24 Python: Matplotlib
-
Matplotlib
is a comprehensive library for creating -
The package is integrated with other third party packages
24.1 Matplotlib
: Overview
Matplotlib
is a comprehensive library for creating static, animated, and interactive visualizations in PythonMatplotlib
can create high-quality plots and interactive figuresMany components of
Matplotlib
are similar to Rbase
plot, although implementation is differentIt is integrated with
pandas
and many third party packagesSee the previous chapter for
pandas
implementation ofMatplotlib
Here we present a typical implementation of
Matplotlib
See Matplotlib tutorials for a comprehensive usage of the package
The link for quick tips and tricks of
Matplotlib
optionsInstall
Matplotlib
fromPyPI
repository:pip install matplotlib
24.2 A Typical Implementation of Matplotlib
24.2.1 Load Libraries & Read Data
Import matplotlib
import matplotlib.pyplot as plt
Import other libraries
import pandas as pd
import numpy as np
Set the working directory to the data folder
Read the iris dataset as a Python object DF
DF = pd.read_csv('iris.csv')
24.2.2 Implementation
# A figure with a single axes
= plt.subplots()
fig, ax
# Create scatter plot
= {'setosa': 'red', 'versicolor': 'blue', 'virginica': 'green'}
colors = DF.Species.unique()
Species = DF.SepalLength, y = DF.PetalLength, marker = 'o', c = DF.Species.map(colors), alpha = 0.5)
ax.scatter(x
# Axes labels
'Sepal Length (cm)')
ax.set_xlabel('Petal Length (cm)');
ax.set_ylabel(
# Legends
# ax.legend()
# Show plot
plt.show()
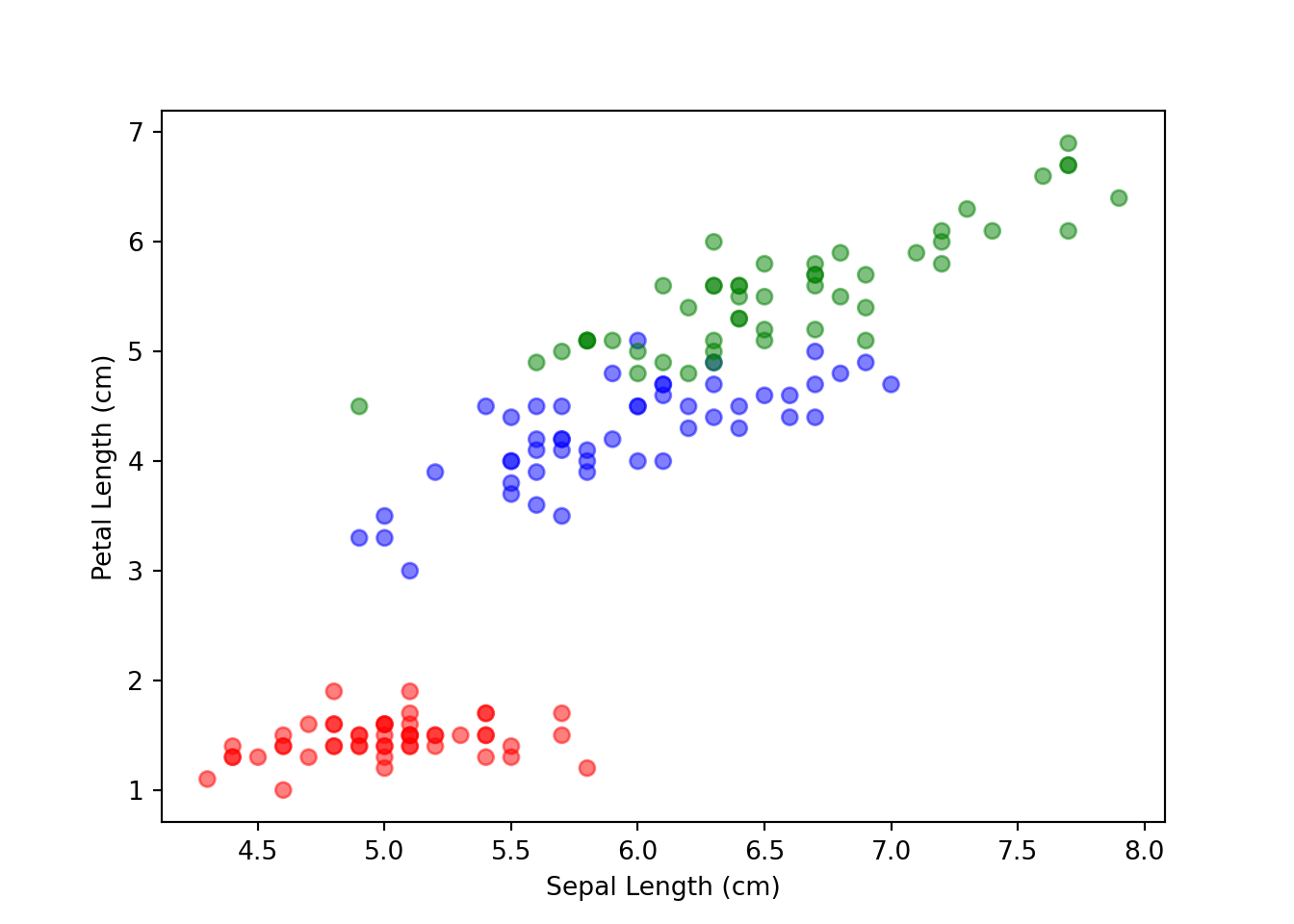
24.3 Components of a Matplotlib Figure
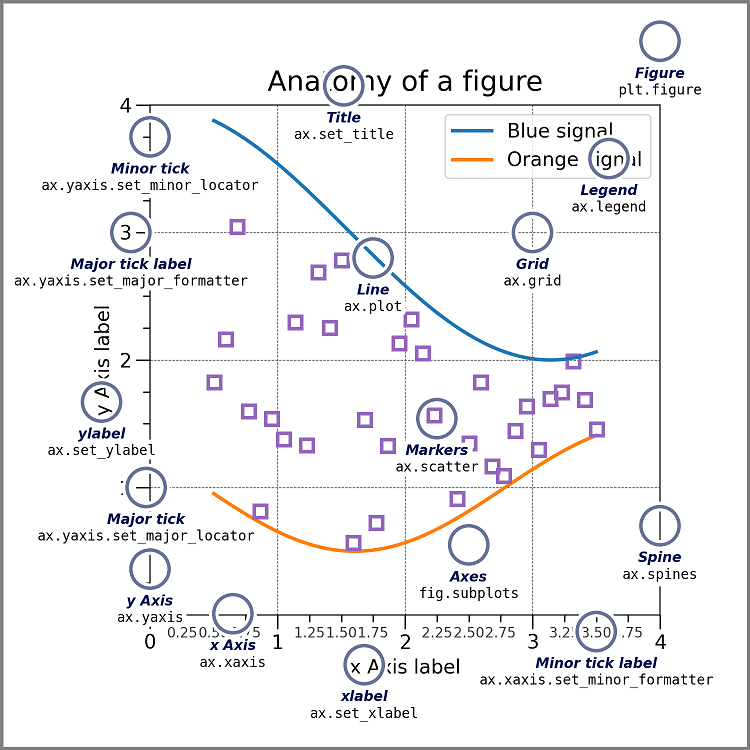
Source: https://matplotlib.org/stable/tutorials/introductory/usage.html